목차
728x90
1.워드 임베딩(Word Embedding)
단어를 저차원의 밀집 벡터(dense vector)로 표현하는 방법
단어를 고차원 희소 표현(Sparse Representation) 방식에서 벗어나 연속적인 실수 값을 가지는 벡터로 변환하여 컴퓨터가 단어 간의 의미적 유사성을 더 잘 파악할 수 있도록 돕는다.
1-1.희소 표현(Sparse Representation)
원-핫 인코딩(One-Hot Encoding)이 대표적이며
데이터나 정보를 표현할 때, 대부분의 값이 0인 벡터나 행렬을 사용하여 표현하는 방식
데이터를 효율적으로 저장하고 처리할 수 있도록 도와준다.
고차원의 데이터를 다룰 때 메모리 소모나 계산 비용이 발생할 수있다.
- "apple" → [1, 0, 0]
- "banana" → [0, 1, 0]
- "cherry" → [0, 0, 1]
1-2.밀집 표현(Dense Representation)
희소 표현(Sparse Representation)에 대조되는 개념
데이터나 정보를 표현할 때 대부분의 값이 0이 아닌 벡터나 행렬을 사용하여 표현하는 방식
데이터에서 거의 모든 값이 의미 있는 정보를 포함하고 있다는 특징을 가지고 있다.
- king [0.23, 0.12, 0.55, ..., -0.34]
- queen [0.24, 0.14, 0.52, ..., -0.35]
- man [0.19, 0.13, 0.53, ..., -0.32]
- woman [0.22, 0.15, 0.51, ..., -0.33]
1-3.분산 표현(Distributed Representation)
데이터나 개체를 고차원 벡터로 표현하는 방법으로, 하나의 단어나 객체를 여러 차원에서의 값으로 분해하여 나타낸다.
즉,하나의 데이터를 여러 차원에 걸쳐 나누어 표현함으로써, 데이터를 더 정교하고 다채롭게 나타낼 수 있다.
각 차원은 해당 데이터의 특성이나 정보를 담고 있고, 비슷한 의미를 가진 데이터는 벡터 공간에서 가까운 위치에 놓이게된다.
2.Word2Vec
고차원 벡터(임베딩)로 변환하는 방법 중 하나로, 단어의 의미적 유사성을 잘 반영할 수 있는 기법이다.
단어 간의 관계나 의미를 벡터 공간에서 수치적으로 표현할 수 있다.
CBOW(Continuous Bag of Words),Skip-gram 방법이 있다.
2-1.CBOW(Continuous Bag of Words)
주변 단어들을 사용하여 중심 단어를 예측하는 방식
문맥(주변 단어들)을 바탕으로 중심 단어를 예측하려고한다.
문장 "The cat sat on the mat"에서
중심 단어를 "sat"라고 한다면, 주변 단어들은 "The", "cat", "on", "the", "mat"이된다.
2-2.Skip-gram
중심 단어를 사용하여 주변 단어들을 예측하는 방식
이 모델은 주어진 중심 단어를 통해 주변 단어들을 예측하려고 학습한다.
문장 "The cat sat on the mat"에서
"sat"라는 중심 단어를 기반으로 "The", "cat", "on", "the", "mat" 같은 주변 단어들을 예측하려고 학습한다.
3.영어 Word2Vec
Word2Vec를 수행후 파일로 저장해둔다.
import re
import urllib.request
import zipfile
from lxml import etree
from nltk.tokenize import word_tokenize, sent_tokenize
from gensim.models import Word2Vec
### 데이터 다운로드
urllib.request.urlretrieve("https://raw.githubusercontent.com/ukairia777/tensorflow-nlp-tutorial/main/09.%20Word%20Embedding/dataset/ted_en-20160408.xml", filename="ted_en-20160408.xml")
### 전처리
targetXML = open('ted_en-20160408.xml', 'r', encoding='UTF8')
target_text = etree.parse(targetXML)
# Content 부분을 가져옴.
parse_text = '\n'.join(target_text.xpath('//content/text()'))
# 정규 표현식
content_text = re.sub(r'\([^)]*\)', '', parse_text)
# 문장 토큰화
sent_text = sent_tokenize(content_text)
# 구두점을 제거,대문자를 소문자로 변환.
normalized_text = []
for string in sent_text:
tokens = re.sub(r"[^a-z0-9]+", " ", string.lower())
normalized_text.append(tokens)
# 각 문장에 대해서 NLTK를 이용하여 단어 토큰화를 수행.
result = [word_tokenize(sentence) for sentence in normalized_text]
### Word2Vec 훈련
model = Word2Vec(sentences=result, vector_size=100, window=5, min_count=5, workers=4, sg=0)
### 모델 저장
model.wv.save_word2vec_format('eng_w2v') # 모델 저장
print("Word2Vec 모델 저장 완료")
다운받은 파일(모델)을 로드 해서 결과를 확인해본다.
from gensim.models import KeyedVectors
loaded_model = KeyedVectors.load_word2vec_format("eng_w2v") # 모델 로드
while True:
try:
word = input("단어를 입력하세요: ")
if word == "exit": break
output = loaded_model.most_similar(word)
print(output)
except:
print("해당 단어는 모델에 없습니다.")
단어를 입력하세요: word
[('phrase', 0.6949447393417358), ('song', 0.6167640686035156), ('poem', 0.5920985341072083), ('sentence', 0.582897424697876), ('language', 0.5820780992507935), ('dictionary', 0.5814653635025024), ('magic', 0.5726603865623474), ('book', 0.5685563087463379), ('trick', 0.562198281288147), ('metaphor', 0.555813193321228)]
4.한글 Word2Vec
import pandas as pd
import matplotlib.pyplot as plt
import urllib.request
from gensim.models.word2vec import Word2Vec
from konlpy.tag import Okt
from tqdm import tqdm
urllib.request.urlretrieve("https://raw.githubusercontent.com/e9t/nsmc/master/ratings.txt", filename="ratings.txt")
train_data = pd.read_table('ratings.txt')
### 데이터 전처리
# 결측값 제거
train_data = train_data.dropna(how = 'any')
# 정규 표현식
train_data['document'] = train_data['document'].str.replace("[^ㄱ-ㅎㅏ-ㅣ가-힣 ]","", regex=True)
# 불용어
stopwords = ['의','가','이','은','들','는','좀','잘','걍','과','도','를','으로','자','에','와','한','하다']
# 토큰화 작업
okt = Okt()
tokenized_data = []
for sentence in tqdm(train_data['document']):
tokenized_sentence = okt.morphs(sentence, stem=True) # 토큰화
stopwords_removed_sentence = [word for word in tokenized_sentence if not word in stopwords] # 불용어 제거
tokenized_data.append(stopwords_removed_sentence)
### Word2Vec 훈련
model = Word2Vec(sentences = tokenized_data, vector_size = 100, window = 5, min_count = 5, workers = 4, sg = 0)
### 모델 저장
model.wv.save_word2vec_format('kor_w2v') # 모델 저장
print("Word2Vec 모델 저장 완료")
from gensim.models import KeyedVectors
loaded_model = KeyedVectors.load_word2vec_format("kor_w2v") # 모델 로드
while True:
try:
word = input("단어를 입력하세요: ")
if word == "exit": break
output = loaded_model.most_similar(word)
print(output)
except:
print("해당 단어는 모델에 없습니다.")
단어를 입력하세요: 고기
[('햄버거', 0.8834248781204224), ('거저', 0.8822125792503357), ('날로', 0.8600116968154907), ('밥', 0.8571436405181885), ('돼지고기', 0.8559597730636597), ('소금', 0.8484519124031067), ('끓이다', 0.8467195630073547), ('해치다', 0.8308089375495911), ('개밥', 0.8273124098777771), ('자장면', 0.826112687587738)]
728x90
'AI' 카테고리의 다른 글
[AI] 임베딩 벡터 시각화 (0) | 2025.01.04 |
---|---|
[AI]과적합(Overfitting)에 대해 (0) | 2025.01.03 |
[AI] 인공 신경망(Artificial Neural Network) 용어1 (1) | 2024.12.30 |
728x90
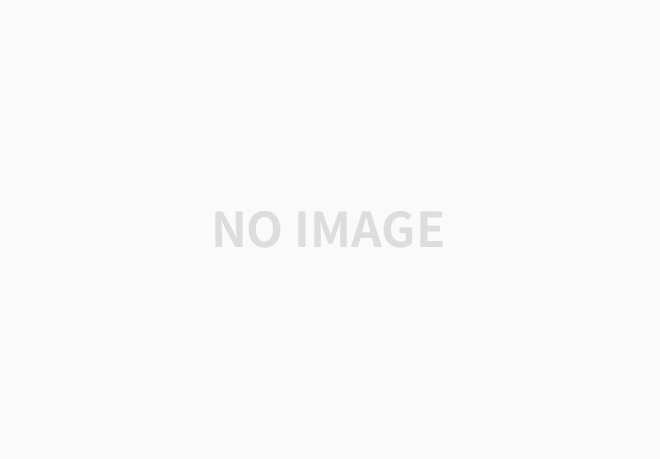
1.워드 임베딩(Word Embedding)
단어를 저차원의 밀집 벡터(dense vector)로 표현하는 방법
단어를 고차원 희소 표현(Sparse Representation) 방식에서 벗어나 연속적인 실수 값을 가지는 벡터로 변환하여 컴퓨터가 단어 간의 의미적 유사성을 더 잘 파악할 수 있도록 돕는다.
1-1.희소 표현(Sparse Representation)
원-핫 인코딩(One-Hot Encoding)이 대표적이며
데이터나 정보를 표현할 때, 대부분의 값이 0인 벡터나 행렬을 사용하여 표현하는 방식
데이터를 효율적으로 저장하고 처리할 수 있도록 도와준다.
고차원의 데이터를 다룰 때 메모리 소모나 계산 비용이 발생할 수있다.
- "apple" → [1, 0, 0]
- "banana" → [0, 1, 0]
- "cherry" → [0, 0, 1]
1-2.밀집 표현(Dense Representation)
희소 표현(Sparse Representation)에 대조되는 개념
데이터나 정보를 표현할 때 대부분의 값이 0이 아닌 벡터나 행렬을 사용하여 표현하는 방식
데이터에서 거의 모든 값이 의미 있는 정보를 포함하고 있다는 특징을 가지고 있다.
- king [0.23, 0.12, 0.55, ..., -0.34]
- queen [0.24, 0.14, 0.52, ..., -0.35]
- man [0.19, 0.13, 0.53, ..., -0.32]
- woman [0.22, 0.15, 0.51, ..., -0.33]
1-3.분산 표현(Distributed Representation)
데이터나 개체를 고차원 벡터로 표현하는 방법으로, 하나의 단어나 객체를 여러 차원에서의 값으로 분해하여 나타낸다.
즉,하나의 데이터를 여러 차원에 걸쳐 나누어 표현함으로써, 데이터를 더 정교하고 다채롭게 나타낼 수 있다.
각 차원은 해당 데이터의 특성이나 정보를 담고 있고, 비슷한 의미를 가진 데이터는 벡터 공간에서 가까운 위치에 놓이게된다.
2.Word2Vec
고차원 벡터(임베딩)로 변환하는 방법 중 하나로, 단어의 의미적 유사성을 잘 반영할 수 있는 기법이다.
단어 간의 관계나 의미를 벡터 공간에서 수치적으로 표현할 수 있다.
CBOW(Continuous Bag of Words),Skip-gram 방법이 있다.
2-1.CBOW(Continuous Bag of Words)
주변 단어들을 사용하여 중심 단어를 예측하는 방식
문맥(주변 단어들)을 바탕으로 중심 단어를 예측하려고한다.
문장 "The cat sat on the mat"에서
중심 단어를 "sat"라고 한다면, 주변 단어들은 "The", "cat", "on", "the", "mat"이된다.
2-2.Skip-gram
중심 단어를 사용하여 주변 단어들을 예측하는 방식
이 모델은 주어진 중심 단어를 통해 주변 단어들을 예측하려고 학습한다.
문장 "The cat sat on the mat"에서
"sat"라는 중심 단어를 기반으로 "The", "cat", "on", "the", "mat" 같은 주변 단어들을 예측하려고 학습한다.
3.영어 Word2Vec
Word2Vec를 수행후 파일로 저장해둔다.
import re
import urllib.request
import zipfile
from lxml import etree
from nltk.tokenize import word_tokenize, sent_tokenize
from gensim.models import Word2Vec
### 데이터 다운로드
urllib.request.urlretrieve("https://raw.githubusercontent.com/ukairia777/tensorflow-nlp-tutorial/main/09.%20Word%20Embedding/dataset/ted_en-20160408.xml", filename="ted_en-20160408.xml")
### 전처리
targetXML = open('ted_en-20160408.xml', 'r', encoding='UTF8')
target_text = etree.parse(targetXML)
# Content 부분을 가져옴.
parse_text = '\n'.join(target_text.xpath('//content/text()'))
# 정규 표현식
content_text = re.sub(r'\([^)]*\)', '', parse_text)
# 문장 토큰화
sent_text = sent_tokenize(content_text)
# 구두점을 제거,대문자를 소문자로 변환.
normalized_text = []
for string in sent_text:
tokens = re.sub(r"[^a-z0-9]+", " ", string.lower())
normalized_text.append(tokens)
# 각 문장에 대해서 NLTK를 이용하여 단어 토큰화를 수행.
result = [word_tokenize(sentence) for sentence in normalized_text]
### Word2Vec 훈련
model = Word2Vec(sentences=result, vector_size=100, window=5, min_count=5, workers=4, sg=0)
### 모델 저장
model.wv.save_word2vec_format('eng_w2v') # 모델 저장
print("Word2Vec 모델 저장 완료")
다운받은 파일(모델)을 로드 해서 결과를 확인해본다.
from gensim.models import KeyedVectors
loaded_model = KeyedVectors.load_word2vec_format("eng_w2v") # 모델 로드
while True:
try:
word = input("단어를 입력하세요: ")
if word == "exit": break
output = loaded_model.most_similar(word)
print(output)
except:
print("해당 단어는 모델에 없습니다.")
단어를 입력하세요: word
[('phrase', 0.6949447393417358), ('song', 0.6167640686035156), ('poem', 0.5920985341072083), ('sentence', 0.582897424697876), ('language', 0.5820780992507935), ('dictionary', 0.5814653635025024), ('magic', 0.5726603865623474), ('book', 0.5685563087463379), ('trick', 0.562198281288147), ('metaphor', 0.555813193321228)]
4.한글 Word2Vec
import pandas as pd
import matplotlib.pyplot as plt
import urllib.request
from gensim.models.word2vec import Word2Vec
from konlpy.tag import Okt
from tqdm import tqdm
urllib.request.urlretrieve("https://raw.githubusercontent.com/e9t/nsmc/master/ratings.txt", filename="ratings.txt")
train_data = pd.read_table('ratings.txt')
### 데이터 전처리
# 결측값 제거
train_data = train_data.dropna(how = 'any')
# 정규 표현식
train_data['document'] = train_data['document'].str.replace("[^ㄱ-ㅎㅏ-ㅣ가-힣 ]","", regex=True)
# 불용어
stopwords = ['의','가','이','은','들','는','좀','잘','걍','과','도','를','으로','자','에','와','한','하다']
# 토큰화 작업
okt = Okt()
tokenized_data = []
for sentence in tqdm(train_data['document']):
tokenized_sentence = okt.morphs(sentence, stem=True) # 토큰화
stopwords_removed_sentence = [word for word in tokenized_sentence if not word in stopwords] # 불용어 제거
tokenized_data.append(stopwords_removed_sentence)
### Word2Vec 훈련
model = Word2Vec(sentences = tokenized_data, vector_size = 100, window = 5, min_count = 5, workers = 4, sg = 0)
### 모델 저장
model.wv.save_word2vec_format('kor_w2v') # 모델 저장
print("Word2Vec 모델 저장 완료")
from gensim.models import KeyedVectors
loaded_model = KeyedVectors.load_word2vec_format("kor_w2v") # 모델 로드
while True:
try:
word = input("단어를 입력하세요: ")
if word == "exit": break
output = loaded_model.most_similar(word)
print(output)
except:
print("해당 단어는 모델에 없습니다.")
단어를 입력하세요: 고기
[('햄버거', 0.8834248781204224), ('거저', 0.8822125792503357), ('날로', 0.8600116968154907), ('밥', 0.8571436405181885), ('돼지고기', 0.8559597730636597), ('소금', 0.8484519124031067), ('끓이다', 0.8467195630073547), ('해치다', 0.8308089375495911), ('개밥', 0.8273124098777771), ('자장면', 0.826112687587738)]
728x90
'AI' 카테고리의 다른 글
[AI] 임베딩 벡터 시각화 (0) | 2025.01.04 |
---|---|
[AI]과적합(Overfitting)에 대해 (0) | 2025.01.03 |
[AI] 인공 신경망(Artificial Neural Network) 용어1 (1) | 2024.12.30 |